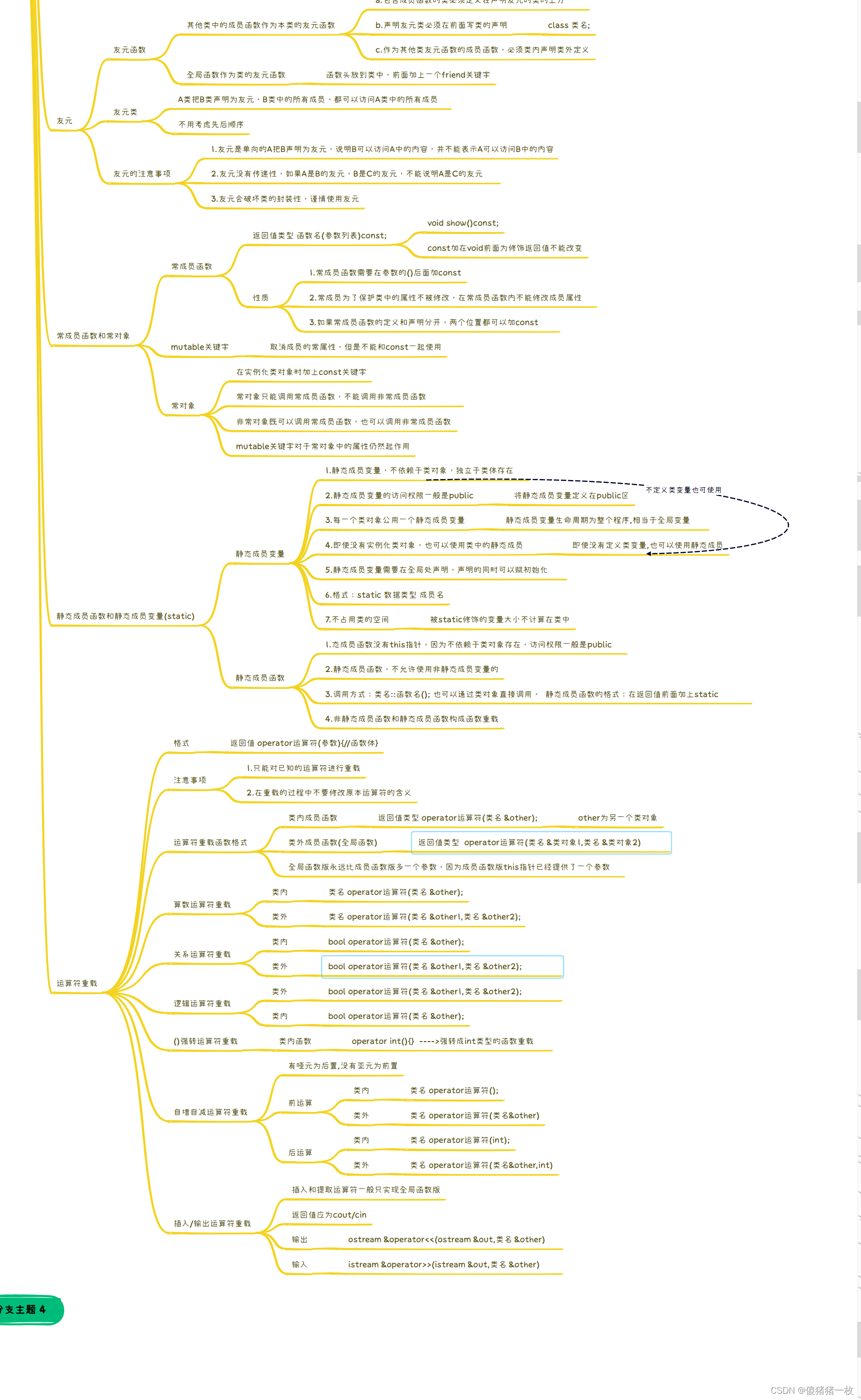
-
定义一个Person类,私有成员int age,string &name,定义一个Stu类,包含私有成员double *score,写出两个类的构造函数、析构函数、拷贝构造和拷贝赋值函数,完成对Person的运算符重载(算术运算符、条件运算符、逻辑运算符、自增自减运算符、插入/提取运算符)
#include <iostream>
using namespace std;
class Person
{
int age;
string &name;
public:
//构造函数
void show();
void show1();
Person(string &a):name(a){}
Person(int a,string &b):age(a),name(b){}
//拷贝构造函数
Person(const Person &other):age(other.age),name(other.name){}
//拷贝赋值函数
Person &operator=(const Person &other)
{
this->age=other.age;
this->name=other.name;
return *(this);
}
//算数运算符
Person operator+(Person &other);//类内定义
friend Person operator+(Person a1,Person a2);//类外定义
Person operator-(Person &other);//类内定义
friend Person operator-(Person a1,Person a2);//类外定义
Person operator*(Person &other);//类内定义
friend Person operator*(Person a1,Person a2);//类外定义
Person operator/(Person &other);//类内定义
friend Person operator/(Person a1,Person a2);//类外定义
Person operator%(Person &other);//类内定义
friend Person operator%(Person a1,Person a2);//类外定义
//条件运算符
bool operator>(Person &other);//类内定义
friend bool operator>(Person a1,Person a2);//类外定义
bool operator>=(Person &other);//类内定义
friend bool operator>=(Person a1,Person a2);//类外定义
bool operator<(Person &other);//类内定义
friend bool operator<(Person a1,Person a2);//类外定义
bool operator<=(Person &other);//类内定义
friend bool operator<=(Person a1,Person a2);//类外定义
bool operator==(Person &other);//类内定义
friend bool operator==(Person a1,Person a2);//类外定义
bool operator!=(Person &other);//类内定义
friend bool operator!=(Person a1,Person a2);//类外定义
//逻辑运算符
bool operator&&(Person &other);//类内定义
friend bool operator&&(Person a1,Person a2);//类外定义
bool operator||(Person &other);//类内定义
friend bool operator||(Person a1,Person a2);//类外定义
//自增自减运算符
Person operator++(int);//类内定义后置++
friend Person operator++(Person &other,int);//类外定义后置++
Person operator++();//类内定义前置++
friend Person operator++(Person &other);//类外定义前置++
Person operator--(int);//类内定义后置++
friend Person operator--(Person &other,int);//类外定义后置--
Person operator--();//类内定义前置--
friend Person operator--(Person &other);//类外定义前置--
//插入提取运算符
friend ostream &operator<<(ostream &out,Person &other);
friend istream &operator>>(istream &in,Person &other);
//析构函数
~Person(){}
};
class Stu
{
double *score;
public:
void show();
//构造函数
Stu(){}
Stu(double score):score(new double(score)){}
//拷贝构造函数
Stu(const Stu& other):score(new double(*other.score)){}
//拷贝赋值函数
Stu &operator=(const Stu &other)
{
*(this->score) = *(other.score);
return (*this);
}
//析构函数
~Stu(){}
};
void Person::show()
{
cout << "age= " << age << endl;
cout << "name=" << name << endl;
}
void Person::show1()
{
cout << "age= " << age << endl;
}
void Stu::show()
{
cout << "*score= " << *score << endl;
}
Person Person::operator+(Person &other)
{
string str("zhangsan");
Person temp(str);
temp.age=this->age+other.age;
//temp.name=this->name+other.name;
return temp;
}
Person operator+(Person a1,Person a2)
{
string str("aaa");
Person temp(str);
temp.age=a1.age+a2.age;
//temp.name=a1.name+a2.name;
return temp;
}
Person Person::operator-(Person &other)
{
string str("zhangsan");
Person temp(str);
temp.age=this->age-other.age;
//temp.name=this->name+other.name;
return temp;
}
Person operator-(Person a1,Person a2)
{
string str("aaa");
Person temp(str);
temp.age=a1.age-a2.age;
//temp.name=a1.name+a2.name;
return temp;
}
Person Person::operator*(Person &other)
{
string str("zhangsan");
Person temp(str);
temp.age=this->age*other.age;
//temp.name=this->name+other.name;
return temp;
}
Person operator*(Person a1,Person a2)
{
string str("aaa");
Person temp(str);
temp.age=a1.age*a2.age;
//temp.name=a1.name+a2.name;
return temp;
}
Person Person::operator/(Person &other)
{
string str("zhangsan");
Person temp(str);
temp.age=this->age/other.age;
//temp.name=this->name+other.name;
return temp;
}
Person operator/(Person a1,Person a2)
{
string str("aaa");
Person temp(str);
temp.age=a1.age/a2.age;
//temp.name=a1.name+a2.name;
return temp;
}
Person Person::operator%(Person &other)
{
string str("zhangsan");
Person temp(str);
temp.age=this->age%other.age;
//temp.name=this->name+other.name;
return temp;
}
Person operator%(Person a1,Person a2)
{
string str("aaa");
Person temp(str);
temp.age=a1.age%a2.age;
//temp.name=a1.name+a2.name;
return temp;
}
bool operator>(Person a1,Person a2)
{
return a1.age > a2.age;
}
bool Person::operator>(Person &a1)
{
return this->age > a1.age;
}
bool operator>=(Person a1,Person a2)
{
return a1.age >= a2.age;
}
bool Person::operator>=(Person &a1)
{
return this->age >= a1.age;
}
bool operator<=(Person a1,Person a2)
{
return a1.age <= a2.age;
}
bool Person::operator<=(Person &a1)
{
return this->age <= a1.age;
}
bool operator<(Person a1,Person a2)
{
return a1.age < a2.age;
}
bool Person::operator<(Person &a1)
{
return this->age < a1.age;
}
bool operator==(Person a1,Person a2)
{
return a1.age == a2.age;
}
bool Person::operator==(Person &a1)
{
return this->age == a1.age;
}
bool operator!=(Person a1,Person a2)
{
return a1.age != a2.age;
}
bool Person::operator!=(Person &a1)
{
return this->age >= a1.age;
}
bool Person::operator&&(Person &other)
{
return this->age && other.age;
}
bool operator&&(Person a1,Person a2)
{
return a1.age && a2.age;
}
bool Person::operator||(Person &other)
{
return this->age || other.age;
}
bool operator||(Person a1,Person a2)
{
return a1.age || a2.age;
}
Person Person::operator++(int)
{
string str="zhangsan";
Person temp(str);
temp.age = this->age++;
return temp;
}
Person operator++(Person &other,int)
{
string str="zhangsan";
Person temp(str);
temp.age=other.age++;
return temp;
}
Person Person::operator++()
{
++(this->age);
return (*this);
}
Person operator++(Person &other)
{
++(other.age);
return other;
}
Person Person::operator--(int)
{
string str="zhangsan";
Person temp(str);
temp.age = this->age--;
return temp;
}
Person operator--(Person &other,int)
{
string str="zhangsan";
Person temp(str);
temp.age=other.age--;
return temp;
}
Person Person::operator--()
{
--(this->age);
return (*this);
}
Person operator--(Person &other)
{
--(other.age);
return other;
}
ostream &operator<<(ostream &out,Person &other)
{
out << other.age << endl;
return out;
}
istream &operator>>(istream &out,Person &other)
{
out >> other.age;
return out;
}
int main()
{
cout << "---person构造函数---" << endl;
string str="shangsan";
Person a1(1,str);
a1.show();
cout << "---person拷贝构造函数---" << endl;
Person a2=a1;
a2.show();
cout << "---person拷贝赋值函数---" << endl;
Person a3(str);
a3=a1;
a3.show();
cout << "---Stu构造函数---" << endl;
Stu b1(100);
b1.show();
cout << "---stu拷贝构造函数---" << endl;
Stu b2=b1;
b2.show();
cout << "---stu拷贝赋值函数---" << endl;
Stu b3;
b3=b1;
b3.show();
string str1("hello");
string str2(" world");
Person a4(10,str1);
Person a5(50,str2);
Person a6=operator+(a4,a5);
a6.show1();
a6=operator-(a4,a5);
a6.show1();
a6=operator*(a4,a5);
a6.show1();
a6=operator/(a4,a5);
a6.show1();
a6=operator%(a4,a5);
a6.show1();
bool c1=operator>(a4,a5);
bool c2=a4.operator>(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator>=(a4,a5);
c2=a4.operator>=(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator<(a4,a5);
c2=a4.operator<(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator<=(a4,a5);
c2=a4.operator<=(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator==(a4,a5);
c2=a4.operator==(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator!=(a4,a5);
c2=a4.operator!=(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
c1=operator&&(a4,a5);
c2=a4.operator&&(a5);
cout << "c1= " << c1 << endl;
cout << "c2= " << c2 << endl;
cout << "aaaa" << endl;
Person a7=a4.operator++();
a7.show1();
a7=operator++(a4);
a7.show1();
a7=a5.operator++(10);
a7.show1();
a7=operator++(a5,10);
a7.show1();
a7=a4.operator--();
a7.show1();
a7=operator--(a4);
a7.show1();
a7=a5.operator--(10);
a7.show1();
a7=operator--(a5,10);
a7.show1();
cout<<a4 << a5 << endl;
operator<<(cout,a4);
operator<<(cout,a5);
Person a8(str);
cin>>a8;
a8.show1();
operator>>(cin,a8);
a8.show1();
return 0;
}