CSharp核心知识点学习
学习内容有:
绪论:面向对象的概念
Lesson1:类和对象
练习:
Lesson2:封装--成员变量和访问修饰符
练习:
Lesson3:封装--成员方法
Lesson4:封装--构造函数和析构函数
知识点四 垃圾回收机制--面试常考
练习:
Lesson5:成员属性
练习:
Lesson6:封装--索引器
练习:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson6_练习
{
#region 练习
//自定义一个整形数组类,该类中有一个整形数组变量
//为它封装增删查改的方法
class IntArray
{
private int[] array;
//房间容量
private int capacity;
//当前放了几个房间
private int length;
public IntArray()
{
capacity = 5;
length = 0;
array = new int[capacity];
}
//增
public void Add(int value)
{
//如果要增加就涉及扩容
//扩容就涉及"搬家"
if(length < capacity)
{
array[length] = value;
++length;
}
//扩容“搬家”
else
{
capacity *= 2;
//新房子
int[] tempArray = new int[capacity];
for (int i = 0; i < array.Length; i++)
{
tempArray[i] = array[i];
}
//老的房子地址 指向新房子地址
array = tempArray;
//传入的往后放
array[length] = value;
++length;
}
}
//删
//指定内容删除
public void Remove(int value)
{
//找到 传入值 在哪个位置
for (int i = 0; i < length; i++)
{
if(array[i] == value)
{
RemoveAt(i);
return;
}
}
Console.WriteLine("没有在数组中找到{0}",value);
}
//指定索引删除
public void RemoveAt(int index)
{
if(index > length)
{
Console.WriteLine("当前数组只有{0},你越界了",length);
return;
}
for (int i = index; i < length - 1; i++)
{
array[i] = array[i + 1];
}
--length;
}
//查改
public int this[int index]
{
get
{
if(index >= length || index < 0)
{
Console.WriteLine("越界了");
return 0;
}
return array[index];
}
set
{
if (index >= length || index < 0)
{
Console.WriteLine("越界了");
}
array[index] = value;
}
}
public int Length
{
get
{
return length;
}
}
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("索引器练习");
IntArray intArray = new IntArray();
intArray.Add(1);
intArray.Add(2);
Console.WriteLine(intArray.Length);
//intArray.RemoveAt(1);
intArray.Remove(2);
Console.WriteLine(intArray[0]);
//Console.WriteLine(intArray[1]);
Console.WriteLine(intArray.Length);
}
}
}
Lesson7:封装--静态成员
练习:
Lesson8:封装-- 静态类和静态构造函数
Lesson9:封装--拓展方法
练习:
Lesson10:封装--运算符重载
练习:
Lesson11:封装--内部类和分部类
Lesson12:继承--继承的基本规则
练习:
Lesson13:继承--里氏替换原则
练习:
Lesson14:继承--继承中的构造函数
练习:
Lesson15:继承--万物之父和装箱拆箱
练习:
Lesson16:继承--密封类
练习:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson16_练习
{
#region 练习
//定义一个载具类
//有速度,最大速度,可乘人数,司机和乘客等,有上车,下车,行驶,车祸等方法
//用载具类申明一个对象,并将若干人装载上车
class Car
{
public int speed;
public int maxSpeed;
//当前装了多少人
public int num;
public Person[] person;
public Car(int speed, int maxSpeed, int num)
{
this.speed = speed;
this.maxSpeed = maxSpeed;
this.num = 0;
person = new Person[num];
}
public void GetIn(Person p)
{
if(num >= person.Length)
{
Console.WriteLine("满载了");
return;
}
person[num] = p;
++num;
}
public void GetOff(Person p)
{
for (int i = 0; i < person.Length; i++)
{
if(person[i] == null)
{
break;
}
if (person[i] == p)
{
//移动位置
for (int j = i; j < num - 1; j++)
{
person[j] = person[j + 1];
}
//最后一个位置清空
person[num - 1] = null;
--num;
break;
}
}
}
public void Move()
{
}
public void Boom()
{
}
}
class Person
{
}
class Driver : Person
{
}
class Passenger : Person
{
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("封装继承综合练习");
Car car = new Car(80, 100, 20);
Driver driver = new Driver();
Passenger p1 = new Passenger();
Passenger p2 = new Passenger();
Passenger p3 = new Passenger();
car.GetIn(driver);
car.GetIn(p1);
car.GetIn(p2);
car.GetIn(p3);
car.GetOff(p1);
}
}
}
练习调试!!!
Lesson17:多态--vob
通过这节课的知识--解决了Lesson13 的练习题
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson13_练习
{
#region 练习一
//is和as的区别
//is 用于判断 类对象判断是不是某个类型的对象 bool
//as 用于转换 把一个类对象 转换成一个指定类型的对象 null
#endregion
#region 练习二
//写一个Monster类,它派生出Boss和Gobin两个类
//Boss有技能,小怪有攻击
//随机生成10个怪,装载到数组中
//遍历这个数组,调用他们的攻击方法,如果是boss就释放技能
class Monster
{
}
class Boss : Monster
{
public void Skill()
{
Console.WriteLine("Boss释放技能");
}
}
class Goblin : Monster
{
public void Atk()
{
Console.WriteLine("小怪攻击");
}
}
#endregion
#region 练习三
//FPS游戏模拟
//写一个玩家类,玩家可以拥有各种武器
//线在有四种武器,冲锋枪,散弹枪,手枪,匕首
//玩家默认拥有匕首
//请在玩家类中写一个方法,可以拾取不同的武器替换自己拥有的武器
class Weapon
{
public virtual string Input()
{
return null;
}
}
/// <summary>
/// 冲锋枪
/// </summary>
class SubmachineGun : Weapon
{
public override string Input()
{
return "冲锋枪";
//Console.WriteLine("冲锋枪");
}
}
/// <summary>
/// 散弹枪
/// </summary>
class ShotGun : Weapon
{
public override string Input()
{
return "散弹枪";
//Console.WriteLine("散弹枪");
}
}
/// <summary>
/// 手枪
/// </summary>
class Pistol : Weapon
{
public override string Input()
{
return "手枪";
//Console.WriteLine("手枪");
}
}
/// <summary>
/// 匕首
/// </summary>
class Dagger : Weapon
{
public override string Input()
{
return "匕首";
//Console.WriteLine("匕首");
}
}
class Player
{
private Weapon nowHaveWeapon;
public Player()
{
nowHaveWeapon = new Dagger();
}
public void PickUp(Weapon weapon)
{
nowHaveWeapon = weapon;
}
public void Show()
{
Console.WriteLine("当前玩家使用的武器是:{0}",nowHaveWeapon.Input());
}
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("里氏替换原则练习");
//随机生成10个怪物
Monster[] objects = new Monster[10];
Random r = new Random();
int box = 0;
for (int i = 0; i < objects.Length; i++)
{
box = r.Next(1, 3);
if(box == 1)
{
objects[i] = new Boss();
}
else
{
objects[i] = new Goblin();
}
}
//遍历数组
for (int i = 0; i < objects.Length; i++)
{
if(objects[i] is Boss)
{
(objects[i] as Boss).Skill();
}
else if(objects[i] is Goblin)
{
(objects[i] as Goblin).Atk();
}
}
Player p = new Player();
p.Show();
Weapon submachineGun = new SubmachineGun();
p.PickUp(submachineGun);
p.Show();
//遗留一个问题:重写?!!
//通过学习Lesson17--多态ovb 得以解决
}
}
}
练习:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson17_练习
{
#region 练习一
//真的鸭子嘎嘎叫,木头鸭子吱吱叫,橡皮鸭子唧唧叫
class Duck
{
public virtual void Speak()
{
Console.WriteLine("嘎嘎叫");
}
}
class WoodDuck : Duck
{
public override void Speak()
{
//base.Speak();
Console.WriteLine("吱吱叫");
}
}
class RubberDuck : Duck
{
public override void Speak()
{
//base.Speak();
Console.WriteLine("唧唧叫");
}
}
#endregion
#region 练习二
//所有员工9点打卡
//但经理十一点打卡,程序员不打卡
class Staff
{
public virtual void PunchTheClock()
{
Console.WriteLine("普通员工9点打卡");
}
}
class Manager : Staff
{
public override void PunchTheClock()
{
//base.PunchTheClock();
Console.WriteLine("经理11点打卡");
}
}
class Programmer : Staff
{
public override void PunchTheClock()
{
//base.PunchTheClock();
Console.WriteLine("程序员不打卡");
}
}
#endregion
#region 练习三
//创建一个图形类,有求面积和周长两个方法
//创建矩形类,正方形类,圆形类继承图形类
//实例化矩形,正方形,圆形对象求面积和周长
class Graph
{
public virtual float GetLength()
{
return 0;
}
public virtual float GetArea()
{
return 0;
}
}
class Rect : Graph
{
private float w;
private float h;
public Rect(float w, float h)
{
this.w = w;
this.h = h;
}
public override float GetLength()
{
//return base.GetLength();
return 2 * (w + h);
}
public override float GetArea()
{
//return base.GetArea();
return w * h;
}
}
class Square : Graph
{
private float h;
public Square(float h)
{
this.h = h;
}
public override float GetLength()
{
//return base.GetLength();
return 4 * h;
}
public override float GetArea()
{
//return base.GetArea();
return h * h;
}
}
class Circule : Graph
{
private float r;
public Circule(float r)
{
this.r = r;
}
public override float GetLength()
{
//return base.GetLength();
return 2 * 3.14F * r;
}
public override float GetArea()
{
//return base.GetArea();
return 3.14f * r * r;
}
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("多态ovb");
Console.WriteLine("virtual override base");
Duck d = new Duck();
d.Speak();
Duck wd = new WoodDuck();
wd.Speak();
Duck rd = new RubberDuck();
rd.Speak();
Staff s = new Staff();
s.PunchTheClock();
Staff ma = new Manager();
ma.PunchTheClock();
(ma as Manager).PunchTheClock();
Staff pr = new Programmer();
(pr as Programmer).PunchTheClock();
Rect r = new Rect(2, 4);
Console.WriteLine("矩形的周长是:" + r.GetLength());
Console.WriteLine("矩形的面积是:" + r.GetArea());
Square sq = new Square(4);
Console.WriteLine("正方形的周长是:" + sq.GetLength());
Console.WriteLine("正方形的面积是:" + sq.GetArea());
Circule ci = new Circule(3);
Console.WriteLine("圆的周长是:" + ci.GetLength());
Console.WriteLine("圆的面积是:" + ci.GetArea());
}
}
}
Lesson18:多态--抽象类和抽象方法
练习:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson18_练习
{
#region 练习一
//写一个动物抽象类,写三个子类
//人叫、狗叫、猫叫
abstract class Animal
{
public abstract void Speak();
}
class Person : Animal
{
public override void Speak()
{
Console.WriteLine("人在说话");
}
}
class Dog : Animal
{
public override void Speak()
{
Console.WriteLine("狗在汪汪叫");
}
}
class Cat : Animal
{
public override void Speak()
{
Console.WriteLine("猫在喵喵叫");
}
}
#endregion
#region 练习二
//创建一个图形类,有求面积和周长两个方法
//创建矩形类,正方形类,圆形类继承图形类
//实例化矩形,正方形,圆形对象求面积和周长
abstract class Graph
{
public abstract float GetLength();
public abstract float GetArea();
}
class Rect : Graph
{
private float w;
private float h;
public Rect(float w, float h)
{
this.w = w;
this.h = h;
}
public override float GetLength()
{
//return base.GetLength();
return 2 * (w + h);
}
public override float GetArea()
{
//return base.GetArea();
return w * h;
}
}
class Square : Graph
{
private float h;
public Square(float h)
{
this.h = h;
}
public override float GetLength()
{
//return base.GetLength();
return 4 * h;
}
public override float GetArea()
{
//return base.GetArea();
return h * h;
}
}
class Circule : Graph
{
private float r;
public Circule(float r)
{
this.r = r;
}
public override float GetLength()
{
//return base.GetLength();
return 2 * 3.14F * r;
}
public override float GetArea()
{
//return base.GetArea();
return 3.14f * r * r;
}
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("抽象类和抽象方法");
Person p = new Person();
p.Speak();
Dog dog = new Dog();
dog.Speak();
Animal cat = new Cat();
cat.Speak();
}
}
}
Lesson19:多态--接口
练习:重要,加深对接口的应用
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Lesson19_练习
{
#region 练习一
//人、汽车、房子都需要登记,人需要到派出所登记,汽车需要去车管所登记,房子需要去房管局登记
//使用接口实现登记方法
interface IRegister
{
void Register();
}
class Person : IRegister
{
public void Register()
{
Console.WriteLine("派出所登记");
}
}
class Car : IRegister
{
public void Register()
{
Console.WriteLine("车管所登记");
}
}
class Home : IRegister
{
public void Register()
{
Console.WriteLine("房管局登记");
}
}
#endregion
#region 练习二
//麻雀、鸵鸟、企鹅、鹦鹉、直升机、天鹅
//直升机和部分鸟能飞
//鸵鸟和企鹅不能飞
//企鹅和天鹅能游泳
//除直升机,其他都能走
//请用面向对象相关知识实现
abstract class Bird
{
public abstract void Walk();
}
interface IFly
{
void Fly();
}
interface ISwimming
{
void Swimming();
}
//麻雀
class Sparrow : Bird,IFly
{
public void Fly()
{
}
public override void Walk()
{
}
}
//鸵鸟
class Ostrich : Bird
{
public override void Walk()
{
}
}
//企鹅
class Penguin : Bird,ISwimming
{
public void Swimming()
{
throw new NotImplementedException();
}
public override void Walk()
{
}
}
//鹦鹉
class Parrot : Bird,IFly
{
public void Fly()
{
throw new NotImplementedException();
}
public override void Walk()
{
}
}
//天鹅
class Swan : Bird,IFly,ISwimming
{
public void Fly()
{
throw new NotImplementedException();
}
public void Swimming()
{
throw new NotImplementedException();
}
public override void Walk()
{
}
}
//直升机
class Helicopter : IFly
{
public void Fly()
{
throw new NotImplementedException();
}
}
#endregion
#region 练习三
//多态来模拟移动硬盘、U盘、MP3插到电脑上读取数据
//移动硬盘与U盘都属于存储设备
//MP3属于播放设备
//但他们都能插在电脑上传输数据
//电脑提供一个USB接口
//请实现电脑的传输数据的功能
interface IUSB
{
void ReadData();
}
class StorageDevice : IUSB
{
public string name;
public StorageDevice(string name)
{
this.name = name;
}
public void ReadData()
{
Console.WriteLine("{0}传输数据",name);
}
}
class MP3 : IUSB
{
public void ReadData()
{
Console.WriteLine("MP3传输数据");
}
}
class Computer
{
public IUSB usb1;
}
#endregion
class Program
{
static void Main(string[] args)
{
Console.WriteLine("接口练习");
IRegister p = new Person();
p.Register();
Car car = new Car();
car.Register();
Home home = new Home();
home.Register();
StorageDevice hd = new StorageDevice("移动硬盘");
StorageDevice ud = new StorageDevice("U盘");
MP3 mp3 = new MP3();
Computer cp = new Computer();
cp.usb1 = hd;
cp.usb1.ReadData();
cp.usb1 = ud;
cp.usb1.ReadData();
cp.usb1 = mp3;
cp.usb1.ReadData();
}
}
}
Lesson20:多态--密封方法
Lesson21:面向对象相关--命名空间
练习:
Lesson22:面向对象相关--万物之父中的方法
练习:
Lesson23:面向对象相关--string
练习:
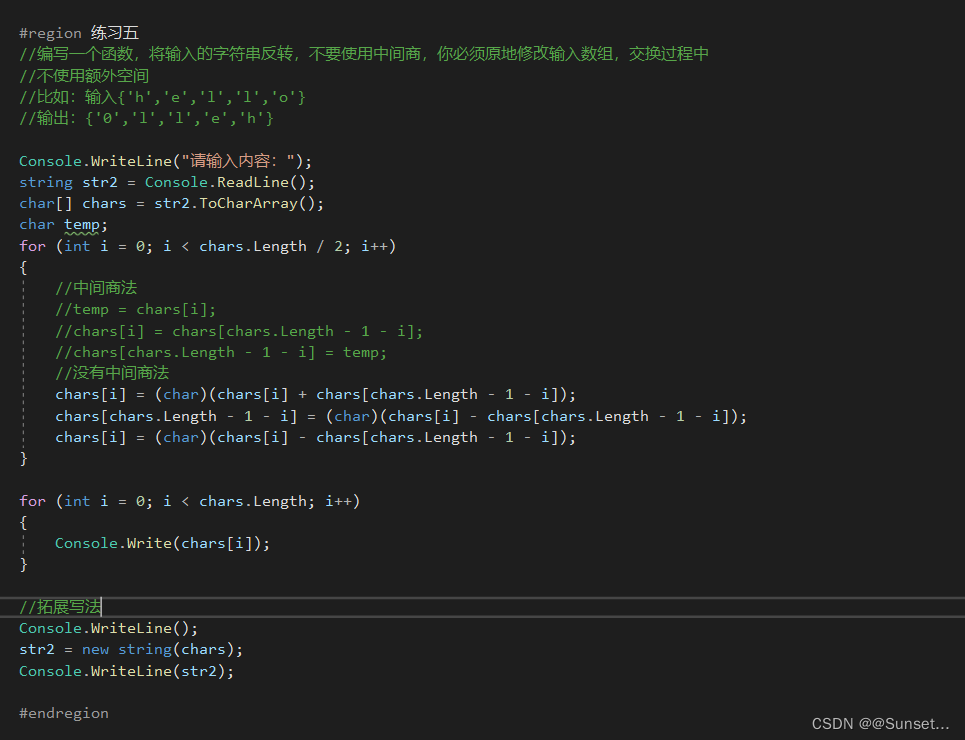
Lesson24:面向对象相关--StringBuilder
练习:
Lesson25:面向对象相关--结构体和类的区别
Lesson26:面向对象相关--抽象类和接口的区别
学习完成:
CSharp核心实践小项目见下一篇笔记!
牢记--多看!!!
你还有好多东西要学习呢,抓紧时间啊!!!