中文版 c++算法入门教程(1)_c++怎么学习算法-CSDN博客
C++is a powerful and widely used programming language, and for those who want to delve deeper into programming and algorithms, mastering C++is an important milestone. This article will take you step by step to understand the basic knowledge of C++programming, and introduce some common algorithms and programming skills to help you get started with C++algorithms.
1. What is Programming?
Programming is the process of using a specific programming language to write a series of instructions or code to tell a computer to perform specific tasks or solve problems. It aims to translate abstract ideas and concepts into instructions that a computer can understand and execute. Programming is used to develop various software applications, websites, games, etc., and allows people to leverage the power of computers for automation, data processing, and innovation.
Here are some important milestones in the development of programming. Interested parties can take a look:
Mechanical computing equipment: In the late 19th and early 20th centuries, a series of mechanical computing equipment emerged, such as differential machines and analytical machines. These mechanical devices require physical settings to perform computational tasks, rather than programming.
Relay and electronic tube: In the mid-20th century, the invention of relays and electronic tubes made computers more reliable and efficient. These devices are capable of performing logical and arithmetic operations, but programming is still done in physical ways (such as patch panels, punched paper tapes).
Binary systems and assembly languages: The popularity of binary systems has enabled computers to represent and process information in digital form. With the introduction of binary systems, assembly language has also emerged. Assembly language uses mnemonics and symbols to represent machine instructions, simplifying the programming process.
Advanced programming languages: In the late 1950s and early 1960s, advanced programming languages began to emerge. These languages (such as Fortran, COBOL, and ALGOL) enable programmers to write code in a more human like manner, thereby improving the readability and maintainability of programming.
Operating System: With the development of computers, the operating system has become a key component for managing computing resources and providing programming interfaces. The operating system enables programmers to use higher-level abstractions to write applications without directly dealing with underlying hardware.
Object oriented programming: In the 1970s and 1980s, object-oriented programming (OOP) became popular. The idea of OOP is to encapsulate data and operations in objects, providing a more flexible and modular programming approach.
Internet and Web programming: With the popularization of the Internet, Web programming has become an important field. Technologies such as HTML, CSS, and JavaScript enable developers to create dynamic and interactive web applications.
The rise of the open source and free software movement has brought a new culture of collaboration and sharing to the programming community. Open source software allows developers to freely access, use, and modify code, promoting innovation and technological progress.
Artificial Intelligence and Machine Learning: In recent years, artificial intelligence and machine learning technologies have made tremendous breakthroughs, bringing new challenges and opportunities to the field of programming. These technologies enable computers to learn and make decisions autonomously from data, driving the development of intelligent systems.
Machine language is binary instruction code that computers can directly understand and execute. Among all programming languages, only machine language can be directly recognized by computers. It is a binary sequence of numbers consisting of a series of 0s and 1s, because computers are electronic devices, in their world there are only negative charges (0) and positive charges (1), used to represent the operations and instructions executed by computer hardware. The machine language of each computer is specific, depending on the processor architecture and instruction set used., Machine language is very low-level and difficult to read and write, but it is the foundation of all other high-level programming languages. A compiler or interpreter for high-level languages translates high-level code into machine language instructions, enabling computers to perform corresponding tasks.
2、 Install Compilation Environment
C++belongs to a type of high-level language. As mentioned above, machine language is the foundation of all other high-level programming languages. High level languages require compilers or interpreters to translate high-level code into machine language instructions in order for computers to perform corresponding tasks. So if you want to learn and use C++, you must install a compiler or interpreter. For beginners learning C++compilers, the following are some suitable choices:
1. Code:: Blocks: Code:: Blocks is a free, open-source, and cross platform integrated development environment (IDE) that uses MinGW as the default C++compiler. It provides a simple and easy-to-use interface and basic debugging functions, making it very suitable for beginners.
2. Dev-C++: Dev-C++is also a free, open-source integrated development environment that uses MinGW as the backend compiler. It provides a concise interface and fast compilation speed, suitable for beginners to do simple C++programming.
3. Visual Studio Community Edition: Visual Studio is a powerful and fully functional IDE, and the Community Edition version is free and suitable for individuals and small teams. It provides rich features, including advanced debugging tools and graphical interface design, suitable for more complex C++projects.
4. Xcode: If you are learning C++on a Mac operating system, Xcode is a great choice. It is a free development tool provided by Apple, with a built-in Clang compiler and debugger, suitable for developing iOS and Mac applications.
I personally believe that when scholars first started learning C++programming, Dev-C++was a good choice. As a free and open-source integrated development environment, Dev-C++provides a simple and intuitive interface, making programming easier to get started with. Dev-C++uses MinGW as the default C++compiler, which has fast compilation speed and good compatibility. Can be found in https://www.123pan.com/s/bZ15Vv-bk9sd.html Download and open the compressed file. Go to the Dev Cpp folder and open devccpp.exe to open the compiler. Of course, you can also download it yourself. After opening the compiler, you will see an interface that may have different colors:
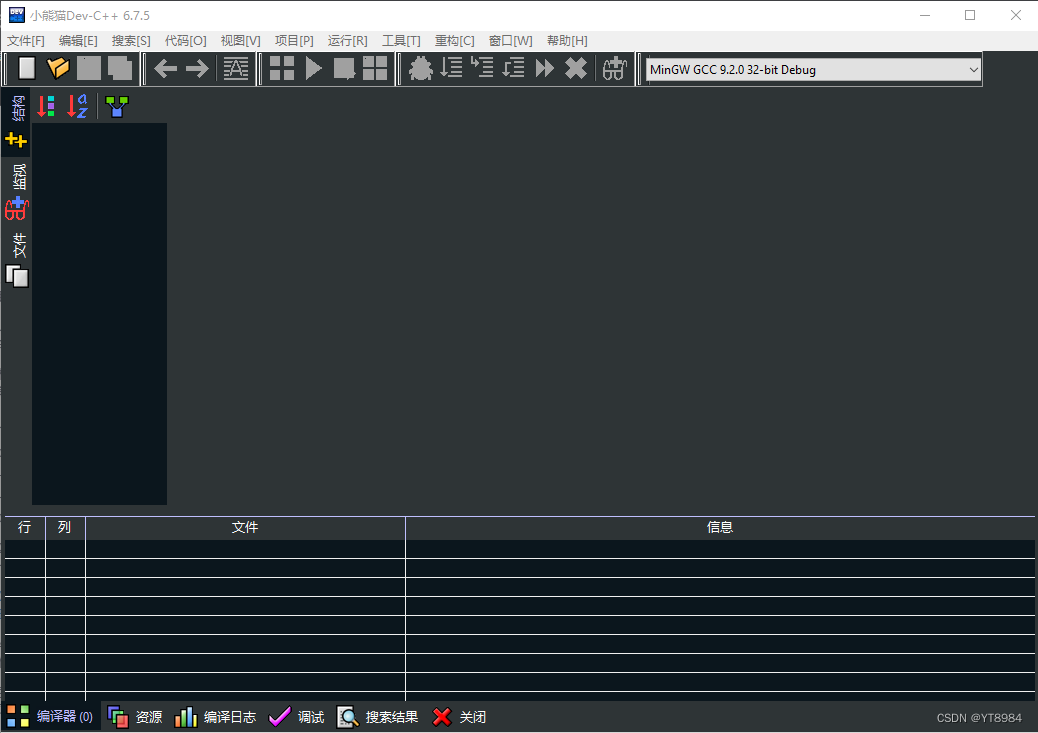
If you want to type code, you need to first create a new file, just follow the steps below and click:
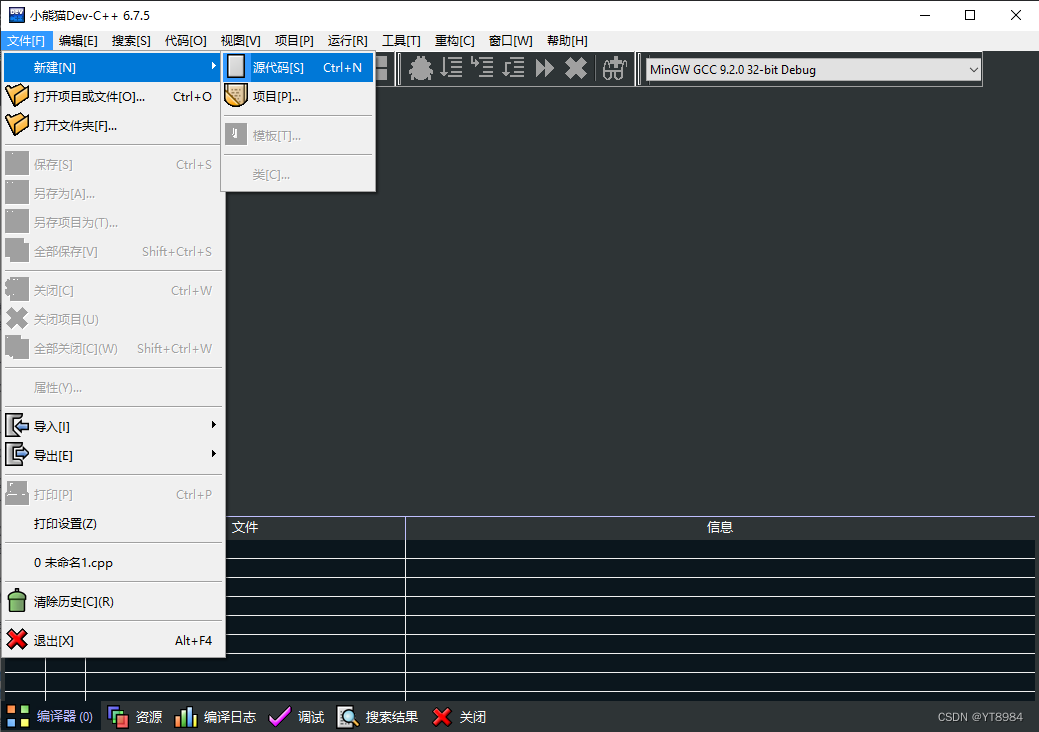
Then you can enter this interface and type the code:
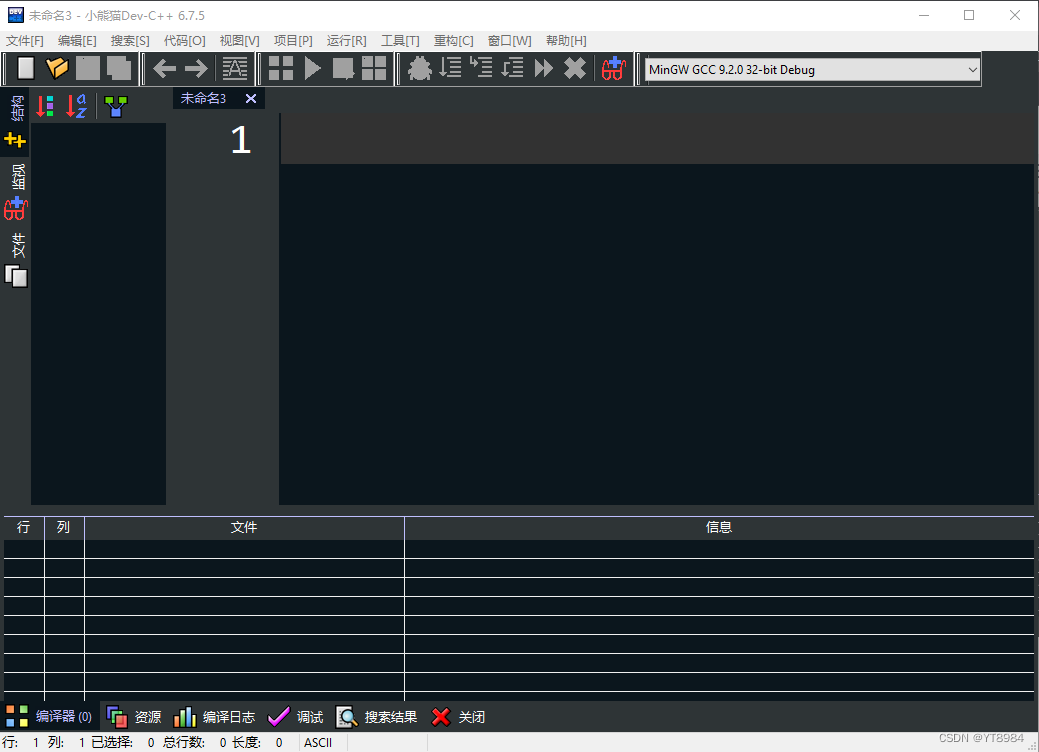
3、 Output
Input usually refers to the external data received by the program. These data can come from users, files, sensors, etc. Input can be various forms of information, such as text, numbers, images, etc. The program uses input data to perform corresponding operations or perform specific calculations.
Output is the result or information returned by the program externally. The output can be text, images, sound, files, etc. It is a product obtained by a program after processing input data, used to display results to users or save results to files or other media. To illustrate with a simple example, let's assume we have written a simple calculator program. The user inputs two numbers and an operator through the keyboard, and the program will perform corresponding operations on the input numbers and output the results to the screen. In this example, the numbers and operators entered by the user are the inputs, while the result obtained by the program after calculation is the output.
In C++, you can use cout to output, which is the standard output stream of C++and can output text to the console. You can use the< For example:
#include <bits/stdc++.h>
using namespace std;
int main() {
cout << "Hello, World!" << endl;//endl是换行
return 0;
}
To run code, press this button:
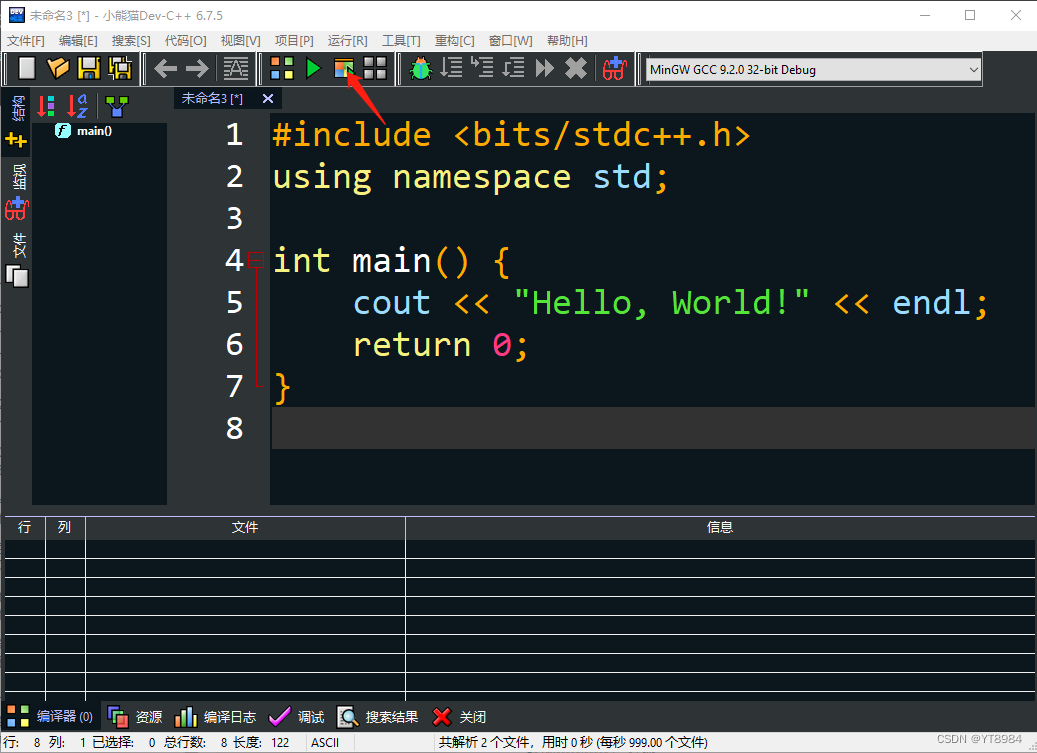
After running, there will be the following effect:
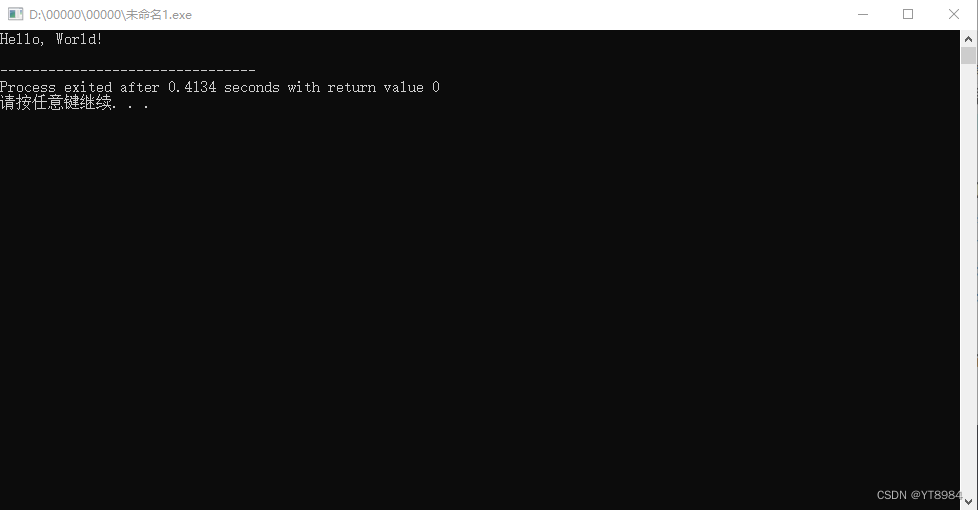
Now you may ask: What kind of code is in the code except for "cout<<" Hello, World! "<
#IncludeAdd a "universal header file". The header file in C++is used to introduce functions, classes, variables, etc. defined in external libraries or other source code files. The header file has an extension of. h or. hpp, usually containing function declarations, macro definitions, structure and class definitions, etc. Simply put, it is to put a library into a program, and there are various types of code in this library for you to use. Different libraries have different code functions, and the universal header file contains the vast majority of commonly used code, so it is called a universal header file, using namespace std; It is a namespace, which is used to prevent function duplication. int main() is the main function in C++programs, and it is the entry point of the program. When the program runs, the operating system will first execute the code in the main function. Return 0; Beginners only need to know that its function refers to closing functions, and; It is a delimiter that must be added after each program segment in C++. Double quotation marks are delimiters used in C++to represent string literals. In the above code, the part enclosed in double quotation marks is "Hello, World!"! "It is a string literal that represents a sequence of characters" Hello, World! "! The string, in simple terms, means that the characters in C++must be enclosed in double quotation marks. If it is only an English character, single quotation marks can be used.
4、 Variables
Variables in C++are containers used to store data. They can be various types, such as integers, floating-point numbers (decimals), characters, strings, etc. In C++, to declare (create) a variable, you need to specify the type and name of the variable. For example, if we want to declare an integer variable, we can use the following syntax:
int n;//int指整数,n指变量名
This will declare an integer variable named n. To assign a value to this variable, the following syntax can be used:
n = 10;
You can also assign values when declaring variables:
int n = 10;
The following are the codes corresponding to different types:
Integer variable (int): used to store integer values. It can store integers in the range of -2147483648 to 2147483647.
Floating point variables (float, double): used to store real values. The float type can store 6-7 significant digits, while the double type can store 15-16 significant digits.
Character type variable (char): used to store a single character.
Boolean variable (bool): used to store Boolean values (true or false 1 or 0).
Short integer variable: used to store smaller integer values. It can store integers in the range of -32768 to 32767.
Long variable: used to store large integer values. It can store integers in the range of -2147483648 to 2147483647.
Unsigned integer variables (unsigned int, unsigned short, unsigned long): used to store positive integer values, excluding negative numbers. The range that can be stored is an integer between 0 and 4294967295.
Long double: A floating-point variable with higher precision than the double type, capable of storing 19-20 significant digits.
Wide character variable (wchar_t): used to store a wide character, usually occupying two or four bytes.
Auto variable: Automatically infer variable type based on initial value.
Register variable: Variables declared as register are stored in CPU registers to speed up program execution.
Extern variable: a global variable defined outside the function and can be used in other files.
Static variables: used to create static variables within a function and globally, which are initialized only once and exist throughout the entire lifecycle of the program.
Const variable: used to represent constants whose values cannot be modified, followed by variable types (int, string...).
5、 Input
If C++wants to input, you can use cin, for example:
#include <bits/stdc++.h>
using namespace std;
int main() {
int n;
cin >> n;
cout << n << endl;
return 0;
}
After running, this is the effect:
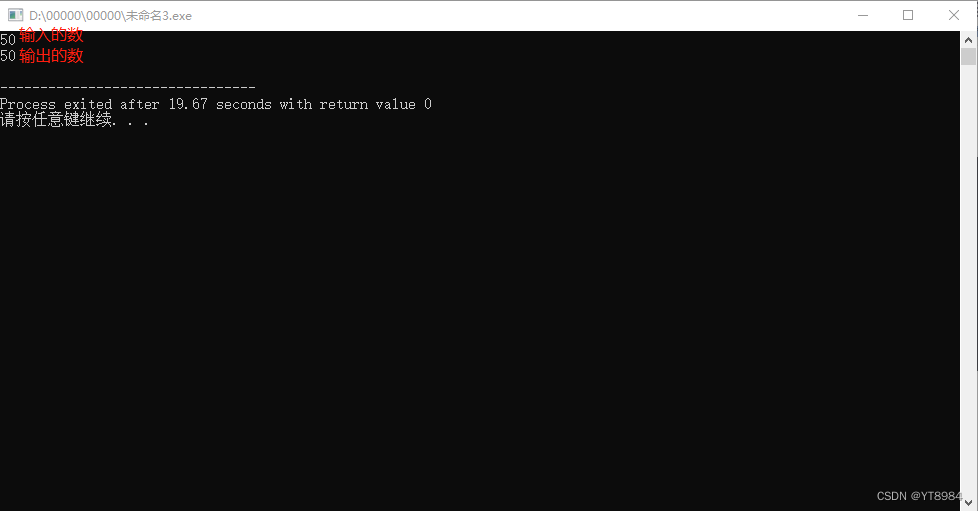
6、 For loop
When scholars first learned C++programming, the for loop was a very important control structure. It is usually used to repeatedly execute a piece of code, allowing the program to iterate according to specified conditions.
The basic syntax of a for loop is as follows:
for (初始化表达式; 条件表达式; 更新表达式) { // 循环体代码 }
Among them:
Initialize expression: Executes before the start of the loop and only once. Usually used to initialize counters or set the initial value of variables.
Conditional expression: Check before each iteration of the loop. If the condition is true (non-zero), continue to execute the loop body; If the condition is false (zero), jump out of the loop.
Update expression: executed after each iteration of the loop, usually used to update counters or change the value of variables.
Here is an example of using a for loop to print numbers 1 to 10:
#include <iostream>
using namespace std;
int main () {
for (int i = 1; i <= 10; i++)
{
cout << i << " ";
}
return 0;
}
In this example, we first initialized counter i with int i=1, and then set the conditional expression i<=10, indicating that as long as i is less than or equal to 10, the loop body will continue to be executed. After each iteration of the loop, counter i will perform an auto increment operation through i++. The code cout in the loop body< Used to output the current counter value i, followed by a space. In this way, the program will output numbers 1 to 10, separated by spaces between each number. The for loop can flexibly modify initialization, conditions, and update expressions according to requirements. You can adjust these parts according to specific programming tasks.