👨💻个人主页:@元宇宙-秩沅
👨💻 hallo 欢迎 点赞👍 收藏⭐ 留言📝 加关注✅!
👨💻 本文由 秩沅 原创
👨💻 收录于专栏:Unity基础实战
⭐🅰️⭐
文章目录
- ⭐🅰️⭐
- ⭐前言⭐
- (==1==)商城系统
- (==2==)背包系统
- (==3==)BOSS系统
⭐前言⭐
(1)商城系统
- 商品逻辑
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using static UnityEditor.Progress;
//------------------------------
//-------功能: 商城物品按钮
//-------创建者:
//------------------------------
public class ShopItem : MonoBehaviour
{
public Button item; //商品按钮
public Text price;
public int priceVaule; //商品价格
public GridLayoutGroup backPack; //购买栏
public int allDamon;
private Vector3 off = new Vector3(1.8f,1,1); //设定物品框的大小
Button btu;
private void Start()
{
item = GetComponent<Button>();
//将价格进行转换
priceVaule = Convert.ToInt32(price.text);
item.onClick.AddListener(()=> //按钮点击事件的添加
{
Debug.Log("现在的水晶为:"+PlayerContorller.GetInstance().damonNum);
Debug.Log("当前的价格为:"+ priceVaule);
//如果钱够了
if ( priceVaule <= PlayerContorller.GetInstance().damonNum)
{
btu = Instantiate(item); //实例化按钮
//减少钻石的数量
Destroy(btu.GetComponent<ShopItem>()); //移除该商品的脚本
PlayerContorller.GetInstance().ReduceDamon(priceVaule, Instantiate(btu));
btu.transform.SetParent(backPack.transform); //移动到购买栏下方
btu.transform.localScale = off;//初始化大小
}
else
{
GameFacade.Instance.SendNotification(PureNotification.SHOW_PANEL,"TipPanel");
}
});
}
private void Update()
{
if (backPack != null)
{
}
}
}
- 面板逻辑
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
//-------------------------------
//-------功能: 商城系统
//-------创建者: -------
//------------------------------
public class StoreView : BasePanel
{
public GridLayoutGroup StoreGrid;
public GridLayoutGroup BackGrid;
public Button backBtu;
public Button bugPack;//放入背包
}
- 面板视图中介(PureMVC)
using PureMVC.Interfaces;
using PureMVC.Patterns.Mediator;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
//-------------------------------
//-------功能: -------
//-------创建者: -------
//------------------------------
public class StoreViewMediator : Mediator
{
//铭牌名
public static string NAME = "StoreViewMediator";
/// <summary>
/// 构造函数
/// </summary>
public StoreViewMediator() : base(NAME)
{
}
/// <summary>
/// 重写监听感兴趣的通知的方法
/// </summary>
/// <returns>返回你需要监听的通知的名字数组</returns>
public override string[] ListNotificationInterests()
{
return new string[] {
};
}
/// <summary>
/// 面板中组件设置(监听相关)
/// </summary>
/// <param name="stateView"></param>
public void setView(StoreView storeView)
{
ViewComponent = storeView;
if(ViewComponent == null) { Debug.Log("面板是空的"); }
storeView.backBtu .onClick.AddListener(()=>
{
SendNotification(PureNotification.HIDE_PANEL, "StorePanel");
});
storeView.bugPack.onClick.AddListener(() =>
{
});
}
/// <summary>
/// 玩家受伤逻辑
/// </summary>
public void Hurt()
{
}
/// <summary>
/// 重写处理通知的方法,处理通知,前提是完成通知的监听
/// </summary>
/// <param name="notification">通知</param>
public override void HandleNotification(INotification notification)
{
switch (notification.Name)
{
}
}
}
(2)背包系统
- 背包系统视图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
//-------------------------------
//-------功能: 背包系统视图
//-------创建者:
//------------------------------
public class BackpackView : BasePanel
{
public Button back; //退出按钮
public GridLayoutGroup grid;
/// <summary>
/// 更新背包中的内容
/// </summary>
/// <param name="itemBtu"></param>
public void AddItem(Button itemBtu)
{
//将传入的按钮设置为布局下面的子物体
itemBtu.transform.SetParent (grid.gameObject.transform );
itemBtu.transform.localScale = Vector3.one ;//初始化商品道具大小
}
}
+ 背包系统视图中介
```csharp
using PureMVC.Interfaces;
using PureMVC.Patterns.Mediator;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
//-------------------------------
//-------功能: 背包面板视图中介
//-------创建者:
//------------------------------
/// <summary>
/// 状态面板视图中介
/// 固定:
/// 1.继承PureMVC的Mediator脚本
/// 2.写构造函数
/// 3.重写监听通知的方法
/// 4.重写处理通知的方法
/// 5.可选:重写注册时的方法
/// </summary>
public class BackpackViewMediator : Mediator
{
//铭牌名
public static string NAME = "BackpackViewMediator";
/// <summary>
/// 构造函数
/// </summary>
public BackpackViewMediator() : base(NAME)
{
}
/// <summary>
/// 重写监听通知的方法,返回需要的监听(通知)
/// </summary>
/// <returns>返回你需要监听的通知的名字数组</returns>
public override string[] ListNotificationInterests()
{
return new string[] {
PureNotification.UPDATA_BACKPACK
//PureNotification.UPDATA_STATE_INFO
};
}
public void SetView(BackpackView backpackView)
{
Debug.Log(backpackView + "执行SetView");
ViewComponent = backpackView;
//开始按钮逻辑监听
backpackView.back.onClick.AddListener(() =>
{
SendNotification(PureNotification.HIDE_PANEL, "BackpackPanel");
});
}
/// <summary>
/// 重写处理通知的方法,处理通知
/// </summary>
/// <param name="notification">通知</param>
public override void HandleNotification(INotification notification)
{
switch (notification.Name)
{
case PureNotification.UPDATA_BACKPACK:
Debug.Log("/执行视图中的放入背包的方法");
//执行视图中的放入背包的方法
(ViewComponent as BackpackView).AddItem(notification.Body as Button);
break;
}
}
}
- 购买后的商品逻辑
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using static UnityEditor.Progress;
//------------------------------
//-------功能: 商城物品按钮
//-------创建者:
//------------------------------
public class ShopItem : MonoBehaviour
{
public Button item; //商品按钮
public Text price;
public int priceVaule; //商品价格
public GridLayoutGroup backPack; //购买栏
public int allDamon;
private Vector3 off = new Vector3(1.8f,1,1); //设定物品框的大小
Button btu;
private void Start()
{
item = GetComponent<Button>();
//将价格进行转换
priceVaule = Convert.ToInt32(price.text);
item.onClick.AddListener(()=> //按钮点击事件的添加
{
Debug.Log("现在的水晶为:"+PlayerContorller.GetInstance().damonNum);
Debug.Log("当前的价格为:"+ priceVaule);
//如果钱够了
if ( priceVaule <= PlayerContorller.GetInstance().damonNum)
{
btu = Instantiate(item); //实例化按钮
//减少钻石的数量
Destroy(btu.GetComponent<ShopItem>()); //移除该商品的脚本
PlayerContorller.GetInstance().ReduceDamon(priceVaule, Instantiate(btu));
btu.transform.SetParent(backPack.transform); //移动到购买栏下方
btu.transform.localScale = off;//初始化大小
}
else
{
GameFacade.Instance.SendNotification(PureNotification.SHOW_PANEL,"TipPanel");
}
});
}
private void Update()
{
if (backPack != null)
{
}
}
}
(3)BOSS系统
- 场景加载
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
//-------------------------------
//-------功能: Boss房间钥匙逻辑
//-------创建者: -------
//------------------------------
public class Key : MonoBehaviour
{
private void OnTriggerStay(Collider other)
{
if (other.gameObject .tag == "Player"&& Input.GetKeyDown(KeyCode.F))
{
SceneManager.LoadScene(1);
}
}
}
- boss攻击
using DG.Tweening;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SocialPlatforms;
using UnityEngine.UI;
//-------------------------------
//-------功能: boss控制器
//-------创建者: -------
//------------------------------
public class BossController : MonoBehaviour
{
public GameObject player; //对标玩家
public Animator animator; //对标动画机
public float hp = 300f; //血量
public Image hpSlider; //血条
private int attack = 30; //敌人的攻击力
public float CD_skill; //技能冷却时间
private void Start()
{
animator = GetComponent<Animator>();
hpSlider = transform.GetChild(0).GetChild(0).GetComponent<Image>();
}
private void Update()
{
CD_skill += Time.deltaTime; //CD一直在累加
}
//碰撞检测
private void OnCollisionStay(Collision collision)
{
if (collision.gameObject.tag == "Player") //检测到如果是玩家的标签
{
Debug.Log("接触到玩家");
if (CD_skill > 3.5f) //攻击动画的冷却时间
{
//触发攻击事件
animator.SetBool("attack", true); //攻击动画激活
EventCenter.GetInstance().EventTrigger(PureNotification.PLAYER_INJURY); //激活玩家受伤事件
//延迟播放动画s
GameFacade.Instance.SendNotification(PureNotification.HURT_UP, attack); //发送玩家受伤扣血的通知
StartCoroutine("delay", collision.gameObject.transform );
CD_skill = 0; //冷却时间归0
}
}
}
IEnumerator delay(Transform transform) //协程迭代器的定义
{
//暂停几秒(协程挂起)
yield return new WaitForSeconds(0.5f);
//暂停两秒后再显示文字
//DOtween
transform.DOMoveZ(transform.localPosition.z - 3, 1); //被撞击Z轴后移
}
//退出 碰撞检测
private void OnCollisionExit(Collision collision)
{
//
if (collision.gameObject.tag == "Player") //检测到如果是玩家的标签
{
Debug.Log("玩家退出");
animator.SetBool("attack", false);
PlayerContorller.GetInstance().animator.SetBool("hurt", false);
}
}
//范围触发检测
private void OnTriggerStay(Collider other)
{
if (other.tag == "Player") //检测到如果是玩家的标签
{
//让怪物看向玩家
transform.LookAt(other.gameObject.transform.position);
animator.SetBool("walk", true);
//并且向其移动
transform.Translate(Vector3.forward * 1 * Time.deltaTime);
}
}
}
⭐<font color=red >🅰️</font>⭐
-
---
⭐[【Unityc#专题篇】之c#进阶篇】](http://t.csdn.cn/wCAYg)
⭐[【Unityc#专题篇】之c#核心篇】](http://t.csdn.cn/wCAYg)
⭐[【Unityc#专题篇】之c#基础篇】](http://t.csdn.cn/4NW3P)
⭐[【Unity-c#专题篇】之c#入门篇】](http://t.csdn.cn/5sJaB)
**⭐**[【Unityc#专题篇】—进阶章题单实践练习](http://t.csdn.cn/ue4Oh)
⭐[【Unityc#专题篇】—基础章题单实践练习](http://t.csdn.cn/czWon)
**⭐**[【Unityc#专题篇】—核心章题单实践练习](http://t.csdn.cn/wCAYg)
---
<font color= red face="仿宋" size=3>**你们的点赞👍 收藏⭐ 留言📝 关注✅是我持续创作,输出优质内容的最大动力!**、
---
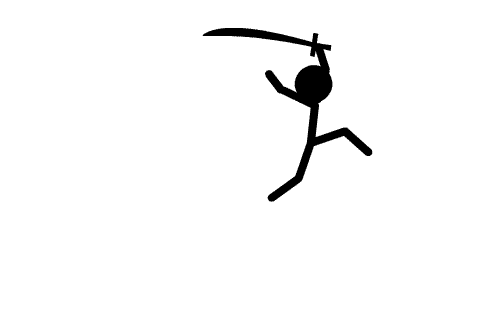
---