一、 使用方法:
在使用前,需要到druid.properties
配置文件中,修改自己对应于自己数据库的属性;如用户名,密码等
driverClassName=com.mysql.cj.jdbc.Driver
url=jdbc:mysql:///hotel?useUnicode=true&characterEncoding=utf8&serverTimezone=UTC
username=root
password=123456
# 初始化连接数量
initialSize=5
# 最大连接数
maxActive=10
# 最大等待时间
maxWait=3000
注意:如果是保持数据库连接异常,则说明数据库和jar包版本匹配了,注意升级数据库jar包版本;
二、项目介绍
- 本项目主要是基于Swing框架搭建的java桌面应用程序 在项目中主要实现的功能有:
1.开始界面
- 开始界面为动态代码雨的欢迎效果
2.注册界面
-
注册界面。实现了验证码的生成和验证,以及出生日期的日历控件化的选择,当用户注册成功后,用户名密码就会通过方法传到登录界面从而避免了用户第二次填账号密码的麻烦
3.分角色登录
-
登录主要分为管理员登录和用户登录,管理员登录后有
- 客房管理功能:这个功能主要实现了对房间的增删改查,以及查看对应房间的评论等;涉及到了多对多的查询
4.用户管理
- 用户管理功能:主要的增删改查操作,点击对应的用户右边小框框展示用户的头像
5.订单管理
- 订单管理功能:在这里会展示用户的全部订单,通过多对多的查询展示用户的订房信息等;已经对用户的一些信息进行统计;主要用到了ifreechart 框架进行绘制表格
6.客房服务
- 客房服务功能:就是给房间添加一些新的设备以及多张配图,方便用户浏览
7.历史记录
- 历史记录:主要记录用户的订房退房记录,实现这个功能主要用到mysql的触发器,通过触发器,没删除一个订单,就将对于的订单保存到历史记录表里边;最后导出表格,而我导出的表格用csv文件逗号阵列,比较方便生成
8.管理员登录
-
管理员管理,主要是设置权限的1位超级管理员0 为普通管理员
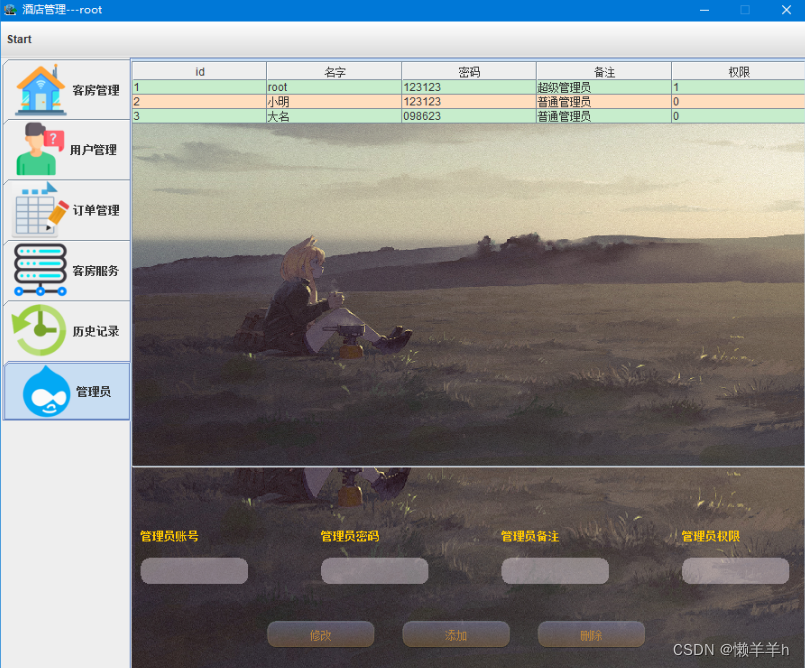
-
在退出前会有监听事件,会询问用户是否最小化托盘,如果最小化托盘则项目已经在运行中
-
在登录前,由于想模仿QQ登录功能输入对应的账号显示不同的头像,最后添加了键盘监听功能和数据库查询,所以刚开始会有点卡
-
9.查看房间
-
用户登录功能
-
用户登录后,可以查看房间和其各种资料;
-
10.点评房间
-
用户也可以点评房间
11.充值界面
-
用户可以对客房进行预订,如果金额不足之前则需要用户进行充值
- 而充值界面需要用户点击我的头像那里进入个人信息页面
-
用户个人信息还有评论记录都可以在个人信息这里修改删除等
三、文件夹目录说明
image 主要用于保存项目中用到的图片文件的
lib 主要用于保存用项目中用到jar包
sql 整个项目运行的数据和数据库表
src 全部源码
com.ludashen.control 主要保存各种自定义的控件的
com.ludashen.dao 数据库交互代码
com.ludashen.frame 用户交互界面代码
com.ludashen.hothl 模型对象类
com.ludashen.panel 面板容器
resource 资源文件,图片等
druid.properties 数据库配置文件
四、 代码
1.runFrame
package com.ludashen.frame;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
public class RunFrame extends JDialog implements ActionListener {
private int gi=0;
private Random random = new Random();
private Dimension screenSize;
private JPanel graphicsPanel;
private final static int gap = 20;
//存放雨点顶部的位置信息(marginTop)
private int[] posArr;
//行数
private int lines;
//列数
private int columns;
public RunFrame() {
initComponents();
}
private void initComponents() {
setLayout(new BorderLayout());
graphicsPanel = new GraphicsPanel();
add(graphicsPanel, BorderLayout.CENTER);
this.setUndecorated(true);
setSize(500,400);
setLocationRelativeTo(null);
this.setDefaultCloseOperation(JDialog.DISPOSE_ON_CLOSE);
setVisible(true);
screenSize = Toolkit.getDefaultToolkit().getScreenSize();
lines = screenSize.height / gap;
columns = screenSize.width / gap;
posArr = new int[columns + 1];
random = new Random();
for (int i = 0; i < posArr.length; i++) {
posArr[i] = random.nextInt(lines);
}
new Timer(120, this).start();
}
private int getChr() {
return random.nextInt(10);
}
//定时器的
@Override
public void actionPerformed(ActionEvent e) {
graphicsPanel.repaint();
}
private class GraphicsPanel extends JPanel {
@Override
public void paint(Graphics g) {
Graphics2D g2d = (Graphics2D) g;
g2d.setFont(getFont().deriveFont(Font.BOLD));
g2d.setColor(Color.BLACK);
g2d.fillRect(0, 0, screenSize.width, screenSize.height);
//当前列
int currentColumn = 0;
for (int x = 0; x < screenSize.width; x += gap) {
int endPos = posArr[currentColumn];
g2d.setColor(Color.CYAN);
g2d.drawString(String.valueOf(getChr()), x, endPos * gap);
int cg = 0;
for (int j = endPos - 15; j < endPos; j++) {
//颜色渐变
cg += 20;
if (cg > 255) {
cg = 255;
}
g2d.setColor(new Color(0, cg, 0));
g2d.drawString(String.valueOf(getChr()), x, j * gap);
}
//每放完一帧,当前列上雨点的位置随机下移1~5行
posArr[currentColumn] += random.nextInt(5);
//当雨点位置超过屏幕高度时,重新产生一个随机位置
if (posArr[currentColumn] * gap > getHeight()) {
posArr[currentColumn] = random.nextInt(lines);
}
currentColumn++;
}
if(gi>6){
g2d.setFont(new Font("italicc",3,25));
g2d.setColor(Color.WHITE);
g2d.drawString("欢迎使用酒店管理系统", getWidth()/2-100, getHeight()/2);
}
if(gi++>25){
dispose();
new LoginFrame().setVisible(true);
}
}
}
public static void main(String[] args) {
new RunFrame();
}
}
2.UserFrame
package com.ludashen.frame;
import com.ludashen.control.*;
import com.ludashen.dao.CommentDao;
import com.ludashen.dao.HouseDao;
import com.ludashen.dao.RoomInfoDao;
import com.ludashen.dao.UserDao;
import com.ludashen.hothl.Comment;
import com.ludashen.hothl.House;
import com.ludashen.hothl.RoomInfo;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class UserFrame extends JFrame {
private Map<String, Object> userInfo;//保存用户信息的
private JMenuBar menuBar;
private JLabel title,img; //选中列表显示房子标题和图片
private JTextPane tDetails; //客房详情
private JTextPane tComment; //顾客点评
private List<House> house;
private int hid; //用于记录房子id的
private String detail; //记录房子详情的
private JList buddyList; //列表信息
private int choose; //获取当前选择的列
private List<String> image=new ArrayList<>();//用于放当前房间的片
private int index;//记录当前放到那张照片了
public UserFrame(String uid){
//构造函数根据登录id查找用户信息
super("酒店管理系统");
try {
userInfo= UserDao.users(uid);
}catch (Exception e){
JOptionPane.showMessageDialog(null,"没有这个用户请重新登录");
System.exit(0);
}
setTitle("酒店系统----"+userInfo.get("uName")+"在线");
init();
}
private void init() {
setResizable(false);
//设置标题图标
setIconImage(Toolkit.getDefaultToolkit().getImage(getClass().getResource("/resource/log.png")));
title= new JLabel();
img=new JLabel();
tDetails=new JTextPane();
tComment=new JTextPane();
tComment.setEnabled(false);
tDetails.setEnabled(false);
tDetails.setBackground(new Color(0x134FAE));
addWindowListener(new WindowAdapter() {
@Override
public void windowClosing(WindowEvent e) {
super.windowClosing(e);
if (JOptionPane.showConfirmDialog(null, "是否最小化托盘") != 0) {
System.exit(0);
}
}
});
tDetails.setContentType("text/html");
tComment.setContentType("text/html");
title.setFont(new Font("",1,30));
topBar();
Tool.SystemTrayInitial(this);
this.add(mainPanel());
this.setSize(900,750);
this.setLocationRelativeTo(null);
}
private JPanel mainPanel(){
JPanel p=new JPanel(null);
JPanel panel = new JPanel();
tDetails.setOpaque(false);
RScrollPane details=new RScrollPane(tDetails,"/resource/bg1.jpg");
panel.setLayout(new BorderLayout());
JPanel p2=new JPanel(new BorderLayout());
JTextField field=new JTextField(50);
field.setSize(500,30);
RButton send=new RButton("发送");
p2.add(field,BorderLayout.WEST);
p2.add(send,BorderLayout.EAST);
panel.add(p2, BorderLayout.SOUTH);
tComment.setOpaque(false);
RScrollPane comment=new RScrollPane(tComment,"/resource/bg1.jpg");
panel.add(comment, BorderLayout.CENTER);
JTabbedPane jTabbedPane =Tool.jTabbedPane(235,450,p,640,215);
jTabbedPane.setTabPlacement(1);
Tool.Tabp(jTabbedPane,"酒店详情", "/resource/tabp/applicatio.png",details, "详情");
Tool.Tabp(jTabbedPane,"点评列表", "/resource/tabp/Comment.png", panel, "列表");
RButton ding=Tool.rButton(800,5,"预定",p,80);
title.setBounds(240,5,260,45);
img.setBounds(240,50,630,400);
p.add(Tool.getFunButton(500, 10, "/resource/button/back1.png", "/resource/button/back2.png", e->{
setBackImage();
}));
p.add(Tool.getFunButton(600, 10, "/resource/button/next1.png", "/resource/button/next2.png", e -> {
setNetImage();
}));
RButton refresh=Tool.rButton(0,633,"刷新",p,235);
send.addActionListener(e->{
Comment comment1=new Comment((String) userInfo.get("uid"),String.valueOf(hid),field.getText());
if(CommentDao.setCommentDao(comment1)) {
tComment.setText(commentDao());
JOptionPane.showMessageDialog(null,"评论成功!");
field.setText("");
}
});
ding.addActionListener(e ->{
new YudingDialog(house.get(choose).getHid(),(String) userInfo.get("uid"),(Float) userInfo.get("money")).setVisible(true);
});
refresh.addActionListener(e -> {
reRoom();
});
p.add(img);
p.add(title);
p.add(listRoom());
p.add(refresh);
return p;
}
private JScrollPane listRoom(){
buddyList = new JList();
buddyList.setOpaque(false);
reRoom();
if(house.size()!=0){
img.setIcon(new ImageIcon((Image) new ImageIcon("image\\room\\"+house.get(0).gethImg()).getImage().getScaledInstance(630, 400,Image.SCALE_DEFAULT )));
title.setText(house.get(0).gethName());
image.add(house.get(0).gethImg());
hid=house.get(0).getHid();
detail= "<html><h2>详情:"+house.get(0).gethDetails()+"</h2>"+roomInfo()+"</html>";
tDetails.setText(detail);
choose=0;
}
else {
tDetails.setText("<h1>没有空房间</h1>");
}
buddyList.addListSelectionListener(e -> {
if(buddyList.getValueIsAdjusting())
try {
img.setIcon(new ImageIcon((Image) new ImageIcon("image\\room\\"+house.get(buddyList.getSelectedIndex()).gethImg()).getImage().getScaledInstance(630, 400,Image.SCALE_DEFAULT ))); //设置JLable的图片
image.clear();
index=0;
image.add(house.get(buddyList.getSelectedIndex()).gethImg());
hid=house.get(buddyList.getSelectedIndex()).getHid();
detail= "<html><h2>详情:"+house.get(buddyList.getSelectedIndex()).gethDetails()+"</h2>"+roomInfo()+"</html>";
title.setText(house.get(buddyList.getSelectedIndex()).gethName());
tDetails.setText(detail);
tComment.setText(commentDao());
choose=buddyList.getSelectedIndex();
for (RoomInfo imgs:RoomInfoDao.getRoomInfo(hid,3)){
image.add(imgs.getFuntion());
}
}catch (Exception e1){
buddyList.clearSelection();
}
});
buddyList.setCellRenderer(new FriListCellRenderer());
buddyList.setFont(new Font(Font.SERIF, Font.PLAIN, 18));
buddyList.setPreferredSize(new Dimension(230, 72*house.size()));
RScrollPane jp = new RScrollPane(buddyList,"");
jp.setBounds(0,0,233,630);
return jp;
}
private void setNetImage() {
if(index==image.size()-1) {
JOptionPane.showMessageDialog(null,"已经是最后一张了");
return;
}
else
index++;
img.setIcon(new ImageIcon((Image) new ImageIcon("image\\room\\"+image.get(index)).getImage().getScaledInstance(630, 400,Image.SCALE_DEFAULT )));
}
private void setBackImage(){
if(index==0) {
JOptionPane.showMessageDialog(null,"已经是第一张了");
return;
}
index--;
img.setIcon(new ImageIcon((Image) new ImageIcon("image\\room\\"+image.get(index)).getImage().getScaledInstance(630, 400,Image.SCALE_DEFAULT )));
}
public void reRoom(){
house= HouseDao.getHouser(2);
HouseModel buddy = new HouseModel(house);
buddyList.setModel(buddy);
}
private String roomInfo(){
List<RoomInfo> roomInfo =RoomInfoDao.getRoomInfo(hid,1);
StringBuffer str=new StringBuffer();
for(RoomInfo info:roomInfo){
str.append("<p>"+info.getName()+":");
str.append(info.getFuntion()+"</p>");
}
return str.toString();
}
private String commentDao(){
int a=0;
List<Comment> comments= CommentDao.getRoomComment(String.valueOf(hid),1);
StringBuffer str=new StringBuffer();
str.append("<html>");
for (Comment comment:comments){
if(a%2==0) {
str.append("<div style='background-color:#3333CC;'><span style='font-size: 20px'>");
str.append(comment.getUid() + "</span><br>");
str.append(comment.getComment() + "<br>" + comment.getDate());
str.append("<br></div>");
}else {
str.append("<div style='background-color:#3300FF;'><span style='font-size: 20px'>");
str.append(comment.getUid() + "</span><br>");
str.append(comment.getComment() + "<br>" + comment.getDate());
str.append("<br></div>");
}
a++;
}
if (comments.size()==0)
str.append("占时没有评论");
str.append("</table></html>");
return str.toString();
}
private void topBar() {
menuBar = new JMenuBar();
menuBar.setLayout(null);
JButton head=new CircleButton(40,(String)userInfo.get("head"));
head.setBounds(840, 0, 40, 40);
head.addMouseListener(new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
super.mouseClicked(e);
new UserInfoDialog(userInfo).setVisible(true);
}
});
JMenu menu = new JMenu("酒店");
menu.setBounds(0,0,80,30);
JMenuItem zmenu = new JMenuItem("酒店简介");
zmenu.addActionListener(e->{
IntroduceDialog introduceDialog = new IntroduceDialog();
introduceDialog.setVisible(true);
new Thread(introduceDialog).start();
});
menu.add(zmenu);
menuBar.add(head);
menuBar.add(menu);
menuBar.setPreferredSize(new Dimension(300, 40));
setJMenuBar(menuBar);
}
}
3.loginFrame
package com.ludashen.frame;
import com.ludashen.control.*;
import com.ludashen.dao.AdminDao;
import com.ludashen.dao.ContFile;
import com.ludashen.dao.UserDao;
import jdk.nashorn.internal.scripts.JO;
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.net.URL;
import java.sql.SQLException;
public class LoginFrame extends JFrame {
private RPanel RP1;
private RPanel RP2;
private JPanel p;
private JTextField userName;
private JPasswordField password;
private static Boolean isAdmin=false;
private JLabel head;
ContFile file=new ContFile();
public LoginFrame(){
super();
init();
}
public static void setAdmin(Boolean b){
isAdmin=b;
}
private void init(){
setResizable(false);
//设置图片
URL re = getClass().getResource("/resource/log.png");
ImageIcon ico =new ImageIcon(re);
setIconImage(Toolkit.getDefaultToolkit().getImage(re));//设置标题图标
this.setLayout(null);
RP1=new RPanel("/resource/Lmain.png");
RP2=new RPanel("/resource/login.png");
RP1.setBounds(0,0,600,400);
RP2.setBounds(0,400,600,100);
RP2.setLayout(null);
RP1.setLayout(null);
Tool.jLabel(50,40,"账号:",RP2);
Tool.jLabel(280,40,"密码:",RP2);
userName= Tool.jTextField(100, 40, RP2,162);
password=Tool.passwordField(330, 40, '*',RP2,159);
head=Tool.jLabel(250,150,"",RP1,100,100);
userName.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
super.keyReleased(e);
head.setIcon(new ImageIcon((Image) new ImageIcon("image\\head\\" +UserDao.head(userName.getText())).getImage().getScaledInstance(100, 100,Image.SCALE_DEFAULT )));
}
});
RButton LoginButton=Tool.rButton(500, 39,"登录",RP2,80);
JLabel register=Tool.jLabel(530, 69,"快速注册",RP2);
JLabel set=Tool.jLabel(480, 69, "设置",RP2);
setChangColor(register, new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
super.mouseClicked(e);
new Register().setVisible(true);
dispose();
}
});
setChangColor(set, new MouseAdapter() {
@Override
public void mouseClicked(MouseEvent e) {
super.mouseClicked(e);
new LoginSetDialog().setVisible(true);
}
});
RP1.add(Tool.getFunButton(560, 5, "/resource/close2.png", "/resource/close1.png", e->{
System.exit(0);
}));
RP1.add(Tool.getFunButton(520, 5, "/resource/min1.png", "/resource/min2.png", e -> {
setExtendedState(JFrame.ICONIFIED);
}));
LoginButton.addActionListener(e -> {
try {
String pass = file.readFile("pass");
String[] sp = pass.split("@");
String name = userName.getText();
String spass = new String(password.getPassword());
if (!isAdmin) {
if (UserDao.getUser(name, spass)) {
if (sp[0].trim().equals("0")) {
file.writeFile("pass", "0@" + name + "@" + spass);
} else {
file.writeFile("pass", "1");
}
new UserFrame(name).setVisible(true);
dispose();
} else {
JOptionPane.showMessageDialog(null, "您现在正在登陆用户系统,而用户密码或名字错误");
}
} else {
if (AdminDao.getAdminLogin(name, spass)) {
if (sp[0].trim().equals("0")) {
file.writeFile("pass", "0@" + name + "@" + spass);
} else {
file.writeFile("pass", "1");
}
new AdminFrame(name).setVisible(true);
dispose();
} else {
JOptionPane.showMessageDialog(null, "您现在正在登陆管理系统,用户密码或名字错误");
}
}
}catch (Exception e1){
// new ContFile().writeFile("e.txt",e1.printStackTrace());
JOptionPane.showMessageDialog(null,e1.getLocalizedMessage());
}
});
pass();
this.add(RP1);
this.add(RP2);
this.setUndecorated(true);//去掉标题栏
this.setSize(600,500);
this.setLocationRelativeTo(null);
this.setDefaultCloseOperation(3);
}
private void pass(){
String pass=file.readFile("pass");
String[] sp=pass.split("@");
if(sp[0].trim().equals("0")&&sp.length>1){
userName.setText(sp[1]);
password.setText(sp[2]);
head.setIcon(new ImageIcon((Image) new ImageIcon("image\\head\\" +sp[1]).getImage().getScaledInstance(100, 100,Image.SCALE_DEFAULT )));
}
}
private void setChangColor(JLabel jLabel,MouseListener l){
jLabel.addMouseListener(l);
jLabel.addMouseListener(new MouseAdapter() {
@Override
public void mouseEntered(MouseEvent e) {
super.mouseEntered(e);
jLabel.setForeground(Color.WHITE);
}
@Override
public void mouseExited(MouseEvent e) {
super.mouseExited(e);
jLabel.setForeground(Color.ORANGE);
}
});
}
public void setUser(String name,String pass){
userName.setText(name);
password.setText(pass);
}
}
五、联系与交流
扣:969060742 运行视频 完整程序资源 sql 程序资源